The scientific Python package known as Dask provides Dask Arrays: parallel, larger-than-memory, n-dimensional arrays that make use of blocked algorithms. They are analogous to Numpy arrays, but are distributed. These terms are defined below:
- Parallel code uses many or all of the cores on the computer running the code.
- Larger-than-memory refers to algorithms that break up data arrays into small pieces, operate on these pieces in an optimized fashion, and stream data from a storage device. This allows a user or programmer to work with datasets of a size larger than the available memory.
- A blocked algorithm speeds up large computations by converting them into a series of smaller computations.
In this tutorial, we cover the use of Xarray to wrap Dask arrays. By using Dask arrays instead of Numpy arrays in Xarray data objects, it becomes possible to execute analysis code in parallel with much less code and effort.
Learning Objectives¶
- Learn the distinction between eager and lazy execution, and performing both types of execution with Xarray
- Understand key features of Dask Arrays
- Learn to perform operations with Dask Arrays in similar ways to performing operations with NumPy arrays
- Understand the use of Xarray
DataArrays
andDatasets
as “Dask collections”, and the use of top-level Dask functions such asdask.visualize()
on such collections - Understand the ability to use Dask transparently in all built-in Xarray operations
Prerequisites¶
Concepts | Importance | Notes |
---|---|---|
Introduction to NumPy | Necessary | Familiarity with Data Arrays |
Introduction to Xarray | Necessary | Familiarity with Xarray Data Structures |
- Time to learn: 30-40 minutes
Imports¶
For this tutorial, as we are working with Dask, there are a number of Dask packages that must be imported. Also, this is technically an Xarray tutorial, so Xarray and NumPy must also be imported. Finally, the Pythia datasets package is imported, allowing access to the Project Pythia example data library.
import dask
import dask.array as da
import numpy as np
import xarray as xr
from dask.diagnostics import ProgressBar
from dask.utils import format_bytes
from pythia_datasets import DATASETS
/home/runner/micromamba/envs/pythia-book-dev/lib/python3.13/site-packages/pythia_datasets/__init__.py:4: UserWarning: pkg_resources is deprecated as an API. See https://setuptools.pypa.io/en/latest/pkg_resources.html. The pkg_resources package is slated for removal as early as 2025-11-30. Refrain from using this package or pin to Setuptools<81.
from pkg_resources import DistributionNotFound, get_distribution
Blocked algorithms¶
As described above, the definition of “blocked algorithm” is an algorithm that replaces a large operation with many small operations. In the case of datasets, this means that a blocked algorithm separates a dataset into chunks, and performs an operation on each.
As an example of how blocked algorithms work, consider a dataset containing a billion numbers, and assume that the sum of the numbers is needed. Using a non-blocked algorithm, all of the numbers are added in one operation, which is extremely inefficient. However, by using a blocked algorithm, the dataset is broken into chunks. (For the purposes of this example, assume that 1,000 chunks are created, with 1,000,000 numbers each.) The sum of the numbers in each chunk is taken, most likely in parallel, and then each of those sums are summed to obtain the final result.
By using blocked algorithms, we achieve the result, in this case one sum of one billion numbers, through the results of many smaller operations, in this case one thousand sums of one million numbers each. (Also note that each of the one thousand sums must then be summed, making the total number of sums 1,001.) This allows for a much greater degree of parallelism, potentially speeding up the code execution dramatically.
dask.array
contains these algorithms¶
The main object type used in Dask is dask.array
, which implements a subset of the ndarray
(NumPy array) interface. However, unlike ndarray
, dask.array
uses blocked algorithms, which break up the array into smaller arrays, as described above. This allows for the execution of computations on arrays larger than memory, by using parallelism to divide the computation among multiple cores. Dask manages and coordinates blocked algorithms for any given computation by using Dask graphs, which lay out in detail the steps Dask takes to solve a problem. In addition, dask.array
objects, known as Dask Arrays, are lazy; in other words, any computation performed on them is delayed until a specific method is called.
Create a dask.array
object¶
As stated earlier, Dask Arrays are loosely based on NumPy arrays. In the next set of examples, we illustrate the main differences between Dask Arrays and NumPy arrays. In order to illustrate the differences, we must have both a Dask Array object and a NumPy array object. Therefore, this first example creates a 3-D NumPy array of random data:
shape = (600, 200, 200)
arr = np.random.random(shape)
arr
array([[[0.69925555, 0.9809442 , 0.86070209, ..., 0.18290126,
0.72288499, 0.02613485],
[0.55263655, 0.30269016, 0.56036633, ..., 0.37036741,
0.51343421, 0.2470729 ],
[0.75543385, 0.36340681, 0.36807292, ..., 0.21245147,
0.11904075, 0.33030987],
...,
[0.82096863, 0.77438884, 0.68863781, ..., 0.45585457,
0.71367319, 0.48087793],
[0.54752797, 0.4043454 , 0.57491639, ..., 0.04755443,
0.93946253, 0.43953363],
[0.41249612, 0.02396684, 0.08093671, ..., 0.60804407,
0.24227515, 0.04958355]],
[[0.89541172, 0.14303128, 0.6348634 , ..., 0.98361763,
0.41470535, 0.99448741],
[0.40639267, 0.29908181, 0.6365261 , ..., 0.64906063,
0.21031588, 0.58900447],
[0.70846159, 0.75930524, 0.92773912, ..., 0.76992983,
0.39285105, 0.10029088],
...,
[0.22498762, 0.15173336, 0.71046297, ..., 0.0313515 ,
0.49042963, 0.35380589],
[0.91210304, 0.28601627, 0.71697179, ..., 0.97244285,
0.02003056, 0.57273074],
[0.41867925, 0.74649524, 0.61644888, ..., 0.7480821 ,
0.08593475, 0.2283603 ]],
[[0.20591035, 0.61287547, 0.10977965, ..., 0.48271641,
0.19357131, 0.24921236],
[0.02813932, 0.65492364, 0.32809424, ..., 0.24499299,
0.35193309, 0.4529757 ],
[0.64803883, 0.94189305, 0.6000646 , ..., 0.4858134 ,
0.59113169, 0.12417377],
...,
[0.17916435, 0.46303764, 0.72866562, ..., 0.79418663,
0.1872416 , 0.78410655],
[0.7238912 , 0.68052155, 0.43963754, ..., 0.78488239,
0.66936629, 0.84168062],
[0.41844469, 0.61009096, 0.66089956, ..., 0.66174581,
0.60372705, 0.9763211 ]],
...,
[[0.87383897, 0.20010157, 0.90645333, ..., 0.03638955,
0.83228705, 0.21974361],
[0.51406297, 0.76056782, 0.65430357, ..., 0.8302979 ,
0.30985844, 0.59437792],
[0.35060477, 0.44694502, 0.3593901 , ..., 0.44688939,
0.63518295, 0.3169143 ],
...,
[0.23125456, 0.8939155 , 0.56399151, ..., 0.48613442,
0.68114228, 0.64950161],
[0.33526804, 0.48874133, 0.72264543, ..., 0.70333183,
0.24291585, 0.87908944],
[0.56748908, 0.56525431, 0.21872954, ..., 0.07923801,
0.8591593 , 0.47798221]],
[[0.38560699, 0.94966206, 0.18511785, ..., 0.34476684,
0.63527114, 0.97577034],
[0.66491392, 0.72385005, 0.56529964, ..., 0.7602657 ,
0.89491176, 0.81933078],
[0.65766073, 0.38915841, 0.85786027, ..., 0.44208648,
0.89526088, 0.49153196],
...,
[0.57739916, 0.55551407, 0.69781127, ..., 0.13603906,
0.38077928, 0.46268591],
[0.66891705, 0.16591995, 0.9558321 , ..., 0.10157685,
0.9269533 , 0.20746925],
[0.94849083, 0.0815058 , 0.05223452, ..., 0.11857401,
0.94129891, 0.3999961 ]],
[[0.67400988, 0.09744179, 0.55097916, ..., 0.26615436,
0.15627054, 0.7226024 ],
[0.18915385, 0.18057518, 0.24234815, ..., 0.46467075,
0.67025239, 0.683523 ],
[0.27505066, 0.31568999, 0.04659975, ..., 0.62176242,
0.20888855, 0.62819605],
...,
[0.73047181, 0.48813204, 0.37071382, ..., 0.32268165,
0.65399103, 0.52352093],
[0.15934248, 0.63502827, 0.85050163, ..., 0.60333824,
0.9838141 , 0.94155708],
[0.40887495, 0.68082999, 0.11800339, ..., 0.31173209,
0.00995737, 0.96897002]]], shape=(600, 200, 200))
format_bytes(arr.nbytes)
'183.11 MiB'
As shown above, this NumPy array contains about 183 MB of data.
As stated above, we must also create a Dask Array. This next example creates a Dask Array with the same dimension sizes as the existing NumPy array:
darr = da.random.random(shape, chunks=(300, 100, 200))
By specifying values to the chunks
keyword argument, we can specify the array pieces that Dask’s blocked algorithms break the array into; in this case, we specify (300, 100, 200)
.
If you are viewing this page as a Jupyter Notebook, the next Jupyter cell will produce a rich information graphic giving in-depth details about the array and each individual chunk.
darr
The above graphic contains a symbolic representation of the array, including shape
, dtype
, and chunksize
. (Your view may be different, depending on how you are accessing this page.) Notice that there is no data shown for this array; this is because Dask Arrays are lazy, as described above. Before we call a compute method for this array, we first illustrate the structure of a Dask graph. In this example, we show the Dask graph by calling .visualize()
on the array:
darr.visualize()
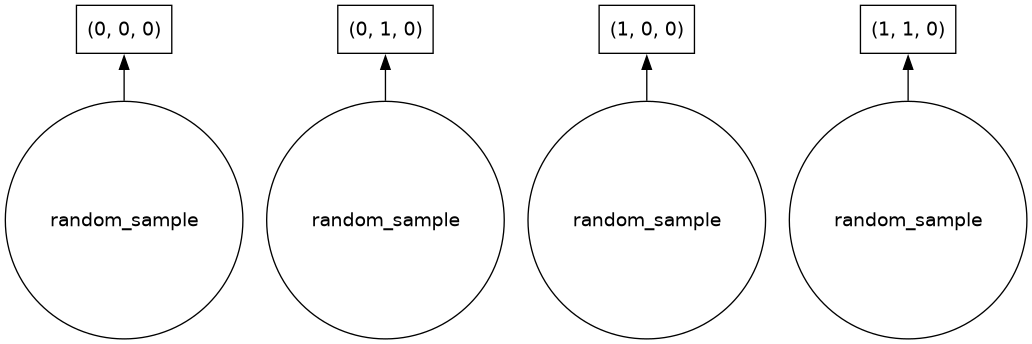
As shown in the above Dask graph, our array has four chunks, each one created by a call to NumPy’s “random” method (np.random.random
). These chunks are concatenated into a single array after the calculation is performed.
Manipulate a dask.array
object as you would a numpy array¶
We can perform computations on the Dask Array created above in a similar fashion to NumPy arrays. These computations include arithmetic, slicing, and reductions, among others.
Although the code for performing these computations is similar between NumPy arrays and Dask Arrays, the process by which they are performed is quite different. For example, it is possible to call sum()
on both a NumPy array and a Dask Array; however, these two sum()
calls are definitely not the same, as shown below.
What’s the difference?¶
When sum()
is called on a Dask Array, the computation is not performed; instead, an expression of the computation is built. The sum()
computation, as well as any other computation methods called on the same Dask Array, are not performed until a specific method (known as a compute method) is called on the array. (This is known as lazy execution.) On the other hand, calling sum()
on a NumPy array performs the calculation immediately; this is known as eager execution.
Why the difference?¶
As described earlier, a Dask Array is divided into chunks. Any computations run on the Dask Array run on each chunk individually. If the result of the computation is obtained before the computation runs through all of the chunks, Dask can stop the computation to save CPU time and memory resources.
This example illustrates calling sum()
on a Dask Array; it also includes a demonstration of lazy execution, as well as another Dask graph display:
total = darr.sum()
total
total.visualize()
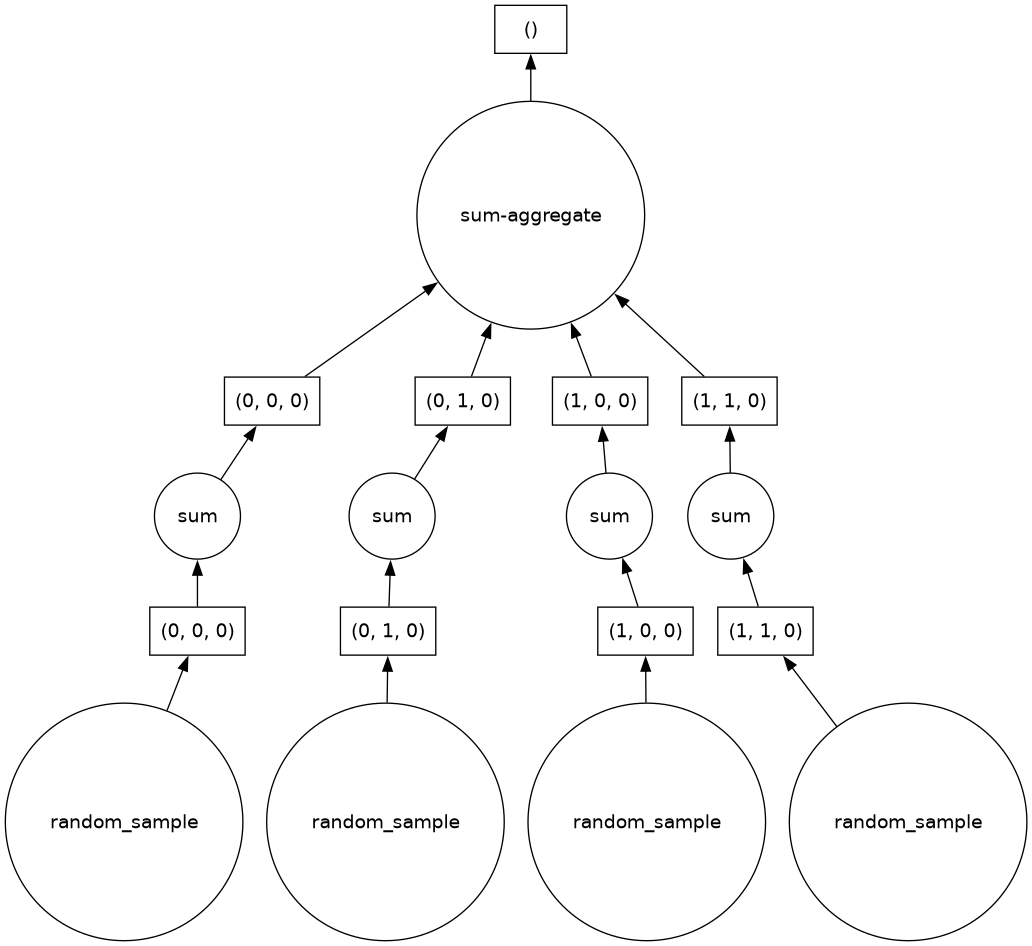
Compute the result¶
As described above, Dask Array objects make use of lazy execution. Therefore, operations performed on a Dask Array wait to execute until a compute method is called. As more operations are queued in this way, the Dask Array’s Dask graph increases in complexity, reflecting the steps Dask will take to perform all of the queued operations.
In this example, we call a compute method, simply called .compute()
, to run on the Dask Array all of the stored computations:
%%time
total.compute()
CPU times: user 389 ms, sys: 46.4 ms, total: 436 ms
Wall time: 901 ms
np.float64(12000481.637928572)
Exercise with dask.arrays
¶
In this section of the page, the examples are hands-on exercises pertaining to Dask Arrays. If these exercises are not interesting to you, this section can be used strictly as examples regardless of how the page is viewed. However, if you wish to participate in the exercises, make sure that you are viewing this page as a Jupyter Notebook.
For the first exercise, modify the chunk size or shape of the Dask Array created earlier. Call .sum()
on the modified Dask Array, and visualize the Dask graph to view the changes.
da.random.random(shape, chunks=(50, 200, 400)).sum().visualize()
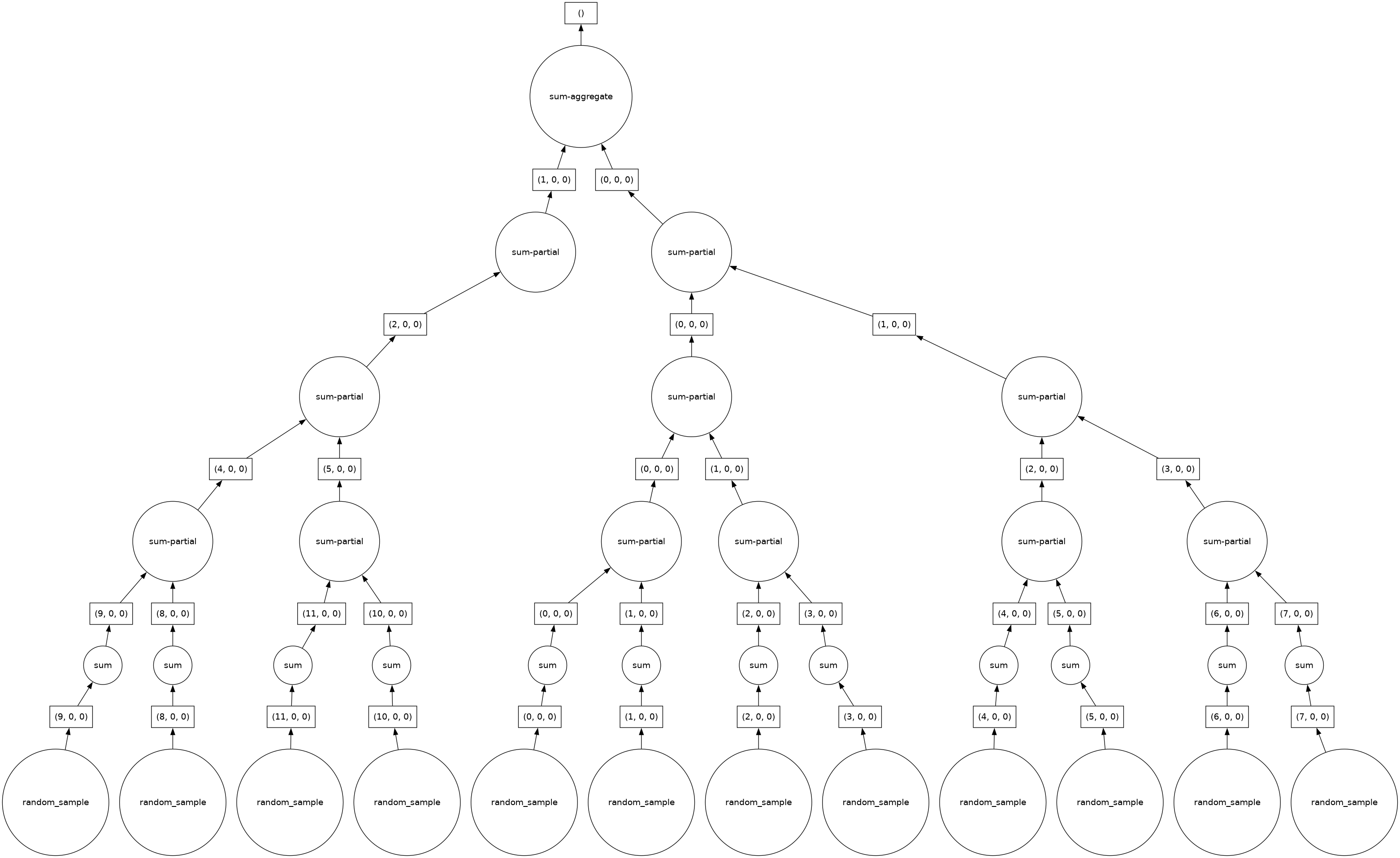
As is obvious from the above exercise, Dask quickly and easily determines a strategy for performing the operations, in this case a sum. This illustrates the appeal of Dask: automatic algorithm generation that scales from simple arithmetic problems to highly complex scientific equations with large datasets and multiple operations.
In this next set of examples, we demonstrate that increasing the complexity of the operations performed also increases the complexity of the Dask graph.
In this example, we use randomly selected functions, arguments and Python slices to create a complex set of operations. We then visualize the Dask graph to illustrate the increased complexity:
z = darr.dot(darr.T).mean(axis=0)[::2, :].std(axis=1)
z
z.visualize()
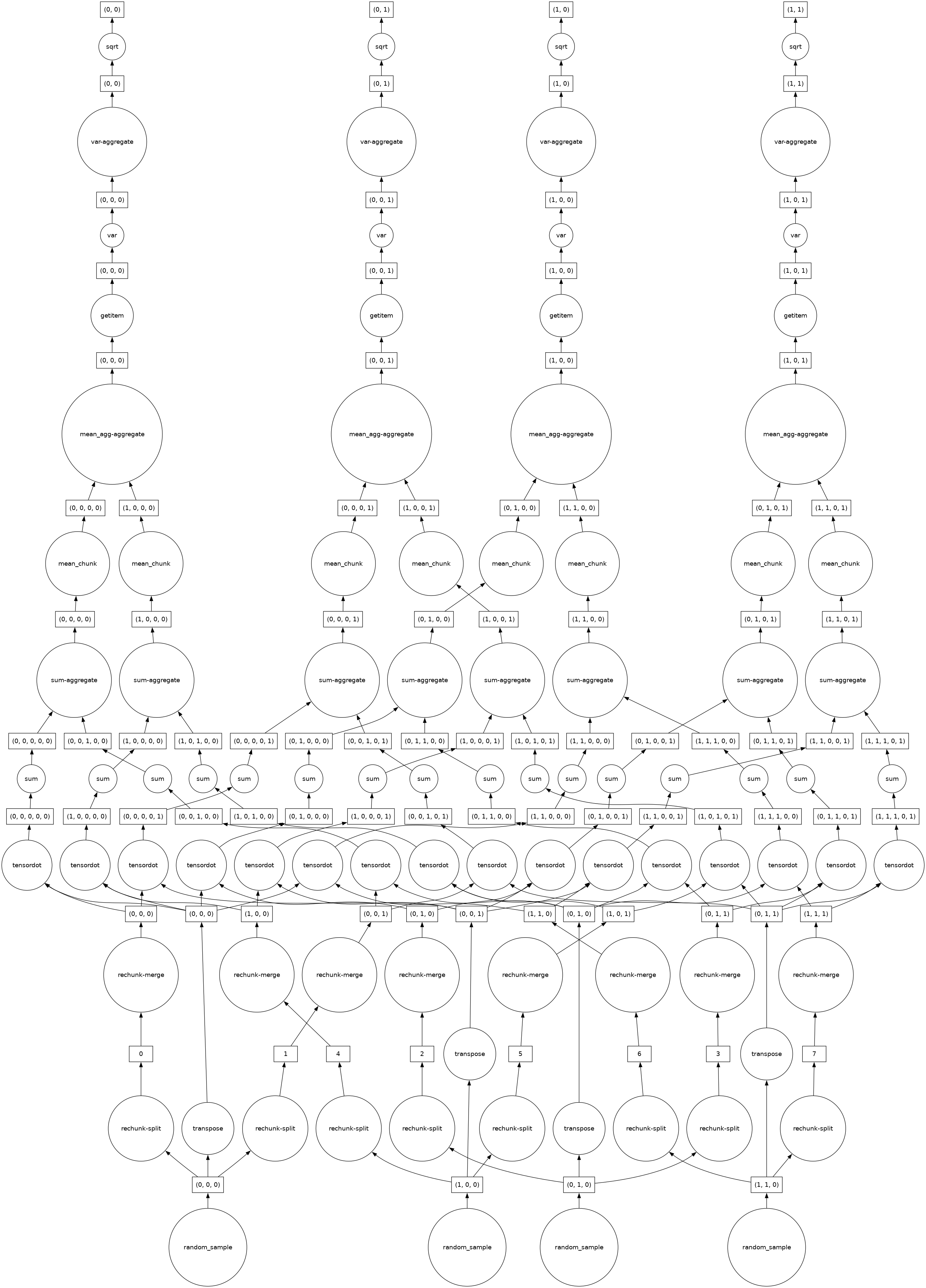
Testing a bigger calculation¶
While the earlier examples in this tutorial described well the basics of Dask, the size of the data in those examples, about 180 MB, is far too small for an actual use of Dask.
In this example, we create a much larger array, more indicative of data actually used in Dask:
darr = da.random.random((4000, 100, 4000), chunks=(1000, 100, 500)).astype('float32')
darr
The dataset created in the previous example is much larger, approximately 6 GB. Depending on how many programs are running on your computer, this may be greater than the amount of free RAM on your computer. However, as Dask is larger-than-memory, the amount of free RAM does not impede Dask’s ability to work on this dataset.
In this example, we again perform randomly selected operations, but this time on the much larger dataset. We also visualize the Dask graph, and then run the compute method. However, as computing complex functions on large datasets is inherently time-consuming, we show a progress bar to track the progress of the computation.
z = (darr + darr.T)[::2, :].mean(axis=2)
z.visualize()

with ProgressBar():
computed_ds = z.compute()
[ ] | 0% Completed | 209.49 us
[ ] | 0% Completed | 103.44 ms
[ ] | 0% Completed | 204.07 ms
[ ] | 0% Completed | 304.80 ms
[ ] | 0% Completed | 410.08 ms
[ ] | 0% Completed | 512.11 ms
[ ] | 0% Completed | 615.09 ms
[ ] | 0% Completed | 716.04 ms
[ ] | 1% Completed | 820.45 ms
[# ] | 3% Completed | 923.13 ms
[## ] | 6% Completed | 1.02 s
[### ] | 9% Completed | 1.13 s
[#### ] | 10% Completed | 1.23 s
[#### ] | 10% Completed | 1.33 s
[#### ] | 10% Completed | 1.43 s
[#### ] | 10% Completed | 1.53 s
[#### ] | 10% Completed | 1.63 s
[#### ] | 10% Completed | 1.74 s
[#### ] | 10% Completed | 1.84 s
[#### ] | 11% Completed | 1.94 s
[##### ] | 13% Completed | 2.04 s
[###### ] | 15% Completed | 2.14 s
[####### ] | 19% Completed | 2.24 s
[######## ] | 21% Completed | 2.35 s
[######## ] | 22% Completed | 2.45 s
[######## ] | 22% Completed | 2.55 s
[######## ] | 22% Completed | 2.65 s
[######## ] | 22% Completed | 2.75 s
[######## ] | 22% Completed | 2.85 s
[######## ] | 22% Completed | 2.96 s
[######## ] | 22% Completed | 3.06 s
[######## ] | 22% Completed | 3.16 s
[######### ] | 24% Completed | 3.26 s
[########## ] | 26% Completed | 3.37 s
[########### ] | 29% Completed | 3.47 s
[############ ] | 31% Completed | 3.57 s
[############# ] | 34% Completed | 3.68 s
[############# ] | 34% Completed | 3.78 s
[############# ] | 34% Completed | 3.88 s
[############# ] | 34% Completed | 3.98 s
[############# ] | 34% Completed | 4.08 s
[############# ] | 34% Completed | 4.19 s
[############# ] | 34% Completed | 4.29 s
[############## ] | 35% Completed | 4.39 s
[############## ] | 36% Completed | 4.49 s
[############### ] | 39% Completed | 4.59 s
[################ ] | 42% Completed | 4.70 s
[################# ] | 44% Completed | 4.80 s
[################## ] | 46% Completed | 4.90 s
[################### ] | 47% Completed | 5.00 s
[################### ] | 47% Completed | 5.10 s
[################### ] | 47% Completed | 5.20 s
[################### ] | 47% Completed | 5.31 s
[################### ] | 47% Completed | 5.41 s
[################### ] | 47% Completed | 5.51 s
[################### ] | 48% Completed | 5.61 s
[#################### ] | 50% Completed | 5.71 s
[#################### ] | 52% Completed | 5.82 s
[###################### ] | 55% Completed | 5.92 s
[####################### ] | 58% Completed | 6.02 s
[####################### ] | 59% Completed | 6.13 s
[####################### ] | 59% Completed | 6.23 s
[####################### ] | 59% Completed | 6.33 s
[####################### ] | 59% Completed | 6.43 s
[####################### ] | 59% Completed | 6.53 s
[####################### ] | 59% Completed | 6.64 s
[####################### ] | 59% Completed | 6.74 s
[######################## ] | 60% Completed | 6.84 s
[######################## ] | 61% Completed | 6.94 s
[######################### ] | 64% Completed | 7.04 s
[########################## ] | 66% Completed | 7.14 s
[########################### ] | 69% Completed | 7.25 s
[############################# ] | 72% Completed | 7.35 s
[############################# ] | 74% Completed | 7.45 s
[############################# ] | 74% Completed | 7.55 s
[############################# ] | 74% Completed | 7.65 s
[############################# ] | 74% Completed | 7.75 s
[############################# ] | 74% Completed | 7.86 s
[############################# ] | 74% Completed | 7.96 s
[############################## ] | 75% Completed | 8.06 s
[############################## ] | 76% Completed | 8.16 s
[############################### ] | 77% Completed | 8.26 s
[############################### ] | 78% Completed | 8.37 s
[################################ ] | 80% Completed | 8.47 s
[################################# ] | 83% Completed | 8.57 s
[################################## ] | 85% Completed | 8.68 s
[################################## ] | 85% Completed | 8.78 s
[################################## ] | 85% Completed | 8.88 s
[################################## ] | 85% Completed | 8.98 s
[################################## ] | 85% Completed | 9.09 s
[################################## ] | 85% Completed | 9.19 s
[################################## ] | 85% Completed | 9.29 s
[################################## ] | 86% Completed | 9.39 s
[################################### ] | 89% Completed | 9.49 s
[#################################### ] | 91% Completed | 9.60 s
[##################################### ] | 94% Completed | 9.70 s
[###################################### ] | 96% Completed | 9.80 s
[########################################] | 100% Completed | 9.90 s
Dask Arrays with Xarray¶
While directly interacting with Dask Arrays can be useful on occasion, more often than not Dask Arrays are interacted with through Xarray. Since Xarray wraps NumPy arrays, and Dask Arrays contain most of the functionality of NumPy arrays, Xarray can also wrap Dask Arrays, allowing anyone with knowledge of Xarray to easily start using the Dask interface.
Reading data with Dask
and Xarray
¶
As demonstrated in previous examples, a Dask Array consists of many smaller arrays, called chunks:
darr
As shown in the following example, to read data into Xarray as Dask Arrays, simply specify the chunks
keyword argument when calling the open_dataset()
function:
ds = xr.open_dataset(DATASETS.fetch('CESM2_sst_data.nc'), chunks={})
ds.tos
While it is a valid operation to pass an empty list to the chunks
keyword argument, this technique does not specify how to chunk the data, and therefore the resulting Dask Array contains only one chunk.
Correct usage of the chunks
keyword argument specifies how many values in each dimension are contained in a single chunk. In this example, specifying the chunks keyword argument as chunks={'time':90}
indicates to Xarray and Dask that 90 time slices are allocated to each chunk on the temporal axis.
Since this dataset contains 180 total time slices, the data variable tos
(holding the sea surface temperature data) is now split into two chunks in the temporal dimension.
ds = xr.open_dataset(
DATASETS.fetch('CESM2_sst_data.nc'),
engine="netcdf4",
chunks={"time": 90, "lat": 180, "lon": 360},
)
ds.tos
It is fairly straightforward to retrieve a list of the chunks and their sizes for each dimension; simply call the .chunks
method on an Xarray DataArray
. In this example, we show that the tos
DataArray
now contains two chunks on the time
dimension, with each chunk containing 90 time slices.
ds.tos.chunks
((90, 90), (180,), (360,))
Xarray data structures are first-class dask collections¶
If an Xarray Dataset
or DataArray
object uses a Dask Array, rather than a NumPy array, it counts as a first-class Dask collection. This means that you can pass such an object to dask.visualize()
and dask.compute()
, in the same way as an individual Dask Array.
In this example, we call dask.visualize
on our Xarray DataArray
, displaying a Dask graph for the DataArray
object:
dask.visualize(ds)
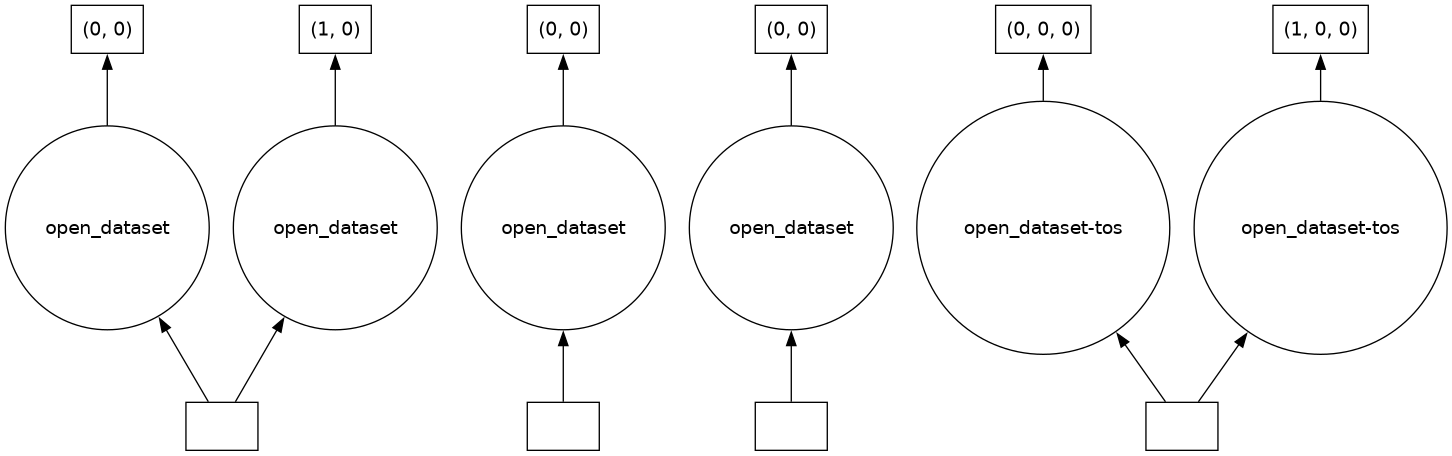
Parallel and lazy computation using dask.array
with Xarray¶
As described above, Xarray Datasets
and DataArrays
containing Dask Arrays are first-class Dask collections. Therefore, computations performed on such objects are deferred until a compute method is called. (This is the definition of lazy computation.)
z = ds.tos.mean(['lat', 'lon']).dot(ds.tos.T)
z
As shown in the above example, the result of the applied operations is an Xarray DataArray
that contains a Dask Array, an identical object type to the object that the operations were performed on. This is true for any operations that can be applied to Xarray DataArrays
, including subsetting operations; this next example illustrates this:
z.isel(lat=0)
Because the data subset created above is also a first-class Dask collection, we can view its Dask graph using the dask.visualize()
function, as shown in this example:
dask.visualize(z)
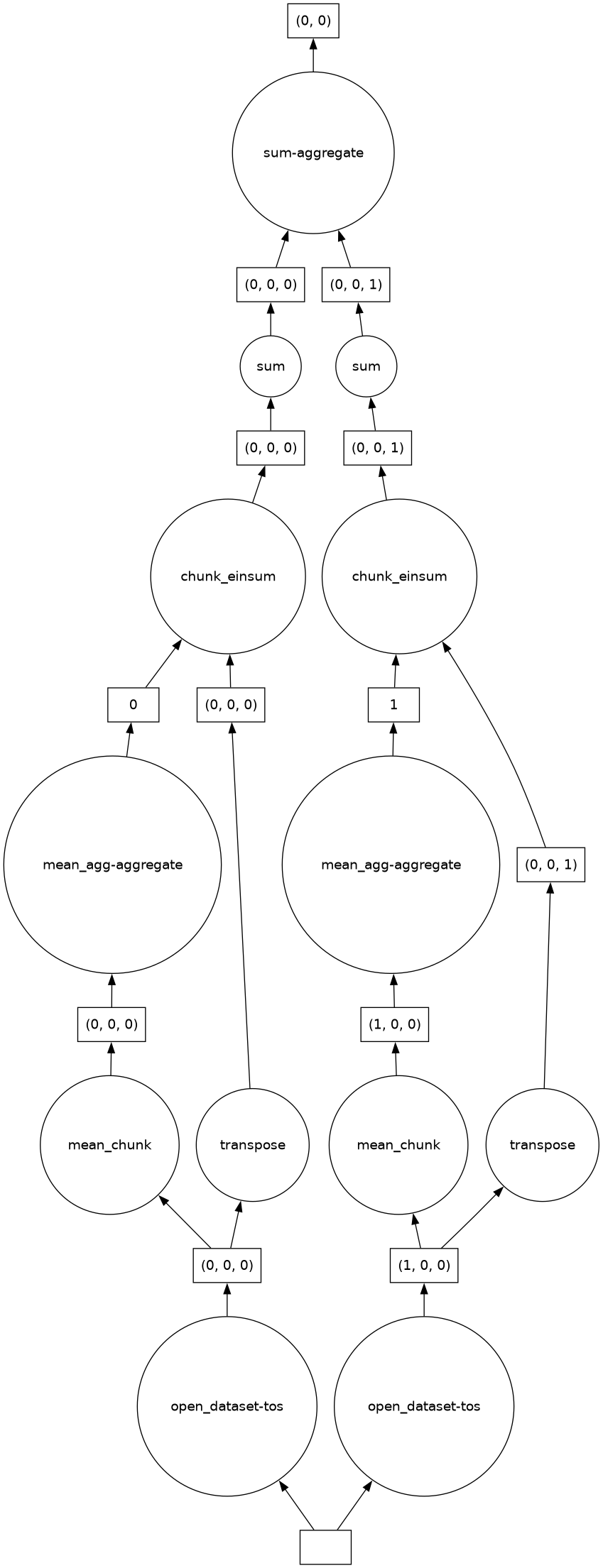
Since this object is a first-class Dask collection, the computations performed on it have been deferred. To run these computations, we must call a compute method, in this case .compute()
. This example also uses a progress bar to track the computation progress.
with ProgressBar():
computed_ds = z.compute()
[ ] | 0% Completed | 475.46 us
[######## ] | 20% Completed | 101.23 ms
[########################################] | 100% Completed | 201.85 ms
Summary¶
This tutorial covered the use of Xarray to access Dask Arrays, and the use of the chunks
keyword argument to open datasets with Dask data instead of NumPy data. Another important concept introduced in this tutorial is the usage of Xarray Datasets
and DataArrays
as Dask collections, allowing Xarray objects to be manipulated in a similar manner to Dask Arrays. Finally, the concepts of larger-than-memory datasets, lazy computation, and parallel computation, and how they relate to Xarray and Dask, were covered.
Dask Shortcomings¶
Although Dask Arrays and NumPy arrays are generally interchangeable, NumPy offers some functionality that is lacking in Dask Arrays. The usage of Dask Array comes with the following relevant issues:
- Operations where the resulting shape depends on the array values can produce erratic behavior, or fail altogether, when used on a Dask Array. If the operation succeeds, the resulting Dask Array will have unknown chunk sizes, which can cause other sections of code to fail.
- Operations that are by nature difficult to parallelize or less useful on very large datasets, such as
sort
, are not included in the Dask Array interface. Some of these operations have supported versions that are inherently more intuitive to parallelize, such astopk
. - Development of new Dask functionality is only initiated when such functionality is required; therefore, some lesser-used NumPy functions, such as
np.sometrue
, are not yet implemented in Dask. However, many of these functions can be added as community contributions, or have already been added in this manner.
Learn More¶
For more in-depth information on Dask Arrays, visit the official documentation page. In addition, this screencast reinforces the concepts covered in this tutorial. (If you are viewing this page as a Jupyter Notebook, the screencast will appear below as an embedded YouTube video.)
from IPython.display import YouTubeVideo
YouTubeVideo(id="9h_61hXCDuI", width=600, height=300)
Resources and references¶
To find specific reference information about Dask and Xarray, see the official documentation pages listed below:
If you require assistance with a specific issue involving Xarray or Dask, the following resources may be of use:
- Dask tag on StackOverflow, for usage questions
- github discussions: dask for general, non-bug, discussion, and usage questions
- github issues: dask for bug reports and feature requests
- github discussions: xarray for general, non-bug, discussion, and usage questions
- github issues: xarray for bug reports and feature requests
Certain sections of this tutorial are adapted from the following existing tutorials: