Quickstart: Zero to Python
Brand new to Python? Here are some quick examples of what Python code looks like.
This is not meant to be a comprehensive Python tutorial, just something to whet your appetite.
Run this code from your browser!
Of course you can simply read through these examples, but it’s more fun to run them yourself:
Find the “Rocket Ship” icon, located near the top-right of this page. Hover over this icon to see the drop-down menu.
Click the
Binder
link from the drop-down menu.This page will open up as a Jupyter notebook in a working Python environment in the cloud.
Press Shift+Enter to execute each code cell
Feel free to make changes and play around!
A very first Python program
A Python program can be a single line:
print("Hello interweb")
Hello interweb
Loops in Python
Let’s start by making a for
loop with some formatted output:
for n in range(3):
print(f"Hello interweb, this is iteration number {n}")
Hello interweb, this is iteration number 0
Hello interweb, this is iteration number 1
Hello interweb, this is iteration number 2
A few things to note:
Python defaults to counting from 0 (like C) rather than from 1 (like Fortran).
Function calls in Python always use parentheses:
print()
The colon
:
denotes the beginning of a definition (here of the repeated code under thefor
loop).Code blocks are identified through indentations.
To emphasize this last point, here is an example with a two-line repeated block:
for n in range(3):
print("Hello interweb!")
print(f"This is iteration number {n}.")
print('And now we are done.')
Hello interweb!
This is iteration number 0.
Hello interweb!
This is iteration number 1.
Hello interweb!
This is iteration number 2.
And now we are done.
Basic flow control
Like most languages, Python has an if
statement for logical decisions:
if n > 2:
print("n is greater than 2!")
else:
print("n is not greater than 2!")
n is not greater than 2!
Python also defines the True
and False
logical constants:
n > 2
False
There’s also a while
statement for conditional looping:
m = 0
while m < 3:
print(f"This is iteration number {m}.")
m += 1
print(m < 3)
This is iteration number 0.
This is iteration number 1.
This is iteration number 2.
False
Basic Python data types
Python is a very flexible language, and many advanced data types are introduced through packages (more on this below). But some of the basic types include:
Integers (int
)
The number m
above is a good example. We can use the built-in function type()
to inspect what we’ve got in memory:
print(type(m))
<class 'int'>
Floating point numbers (float
)
Floats can be entered in decimal notation:
print(type(0.1))
<class 'float'>
or in scientific notation:
print(type(4e7))
<class 'float'>
where 4e7
is the Pythonic representation of the number \( 4 \times 10^7 \).
Character strings (str
)
You can use either single quotes ''
or double quotes " "
to denote a string:
print(type("orange"))
<class 'str'>
print(type('orange'))
<class 'str'>
Lists
A list is an ordered container of objects denoted by square brackets:
mylist = [0, 1, 1, 2, 3, 5, 8]
Lists are useful for lots of reasons including iteration:
for number in mylist:
print(number)
0
1
1
2
3
5
8
Lists do not have to contain all identical types:
myweirdlist = [0, 1, 1, "apple", 4e7]
for item in myweirdlist:
print(type(item))
<class 'int'>
<class 'int'>
<class 'int'>
<class 'str'>
<class 'float'>
This list contains a mix of int
(integer), float
(floating point number), and str
(character string).
Because a list is ordered, we can access items by integer index:
myweirdlist[3]
'apple'
remembering that we start counting from zero!
Python also allows lists to be created dynamically through list comprehension like this:
squares = [i**2 for i in range(11)]
squares
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Dictionaries (dict
)
A dictionary is a collection of labeled objects. Python uses curly braces {}
to create dictionaries:
mypet = {
"name": "Fluffy",
"species": "cat",
"age": 4,
}
type(mypet)
dict
We can then access items in the dictionary by label using square brackets:
mypet["species"]
'cat'
We can iterate through the keys (or labels) of a dict
:
for key in mypet:
print("The key is:", key)
print("The value is:", mypet[key])
The key is: name
The value is: Fluffy
The key is: species
The value is: cat
The key is: age
The value is: 4
Arrays of numbers with NumPy
The vast majority of scientific Python code makes use of packages that extend the base language in many useful ways.
Almost all scientific computing requires ordered arrays of numbers, and fast methods for manipulating them. That’s what NumPy does in the Python world.
Using any package requires an import
statement, and (optionally) a nickname to be used locally, denoted by the keyword as
:
import numpy as np
Now all our calls to numpy
functions will be preceeded by np.
Create a linearly space array of numbers:
# linspace() takes 3 arguments: start, end, total number of points
numbers = np.linspace(0.0, 1.0, 11)
numbers
array([0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1. ])
We’ve just created a new type of object defined by NumPy:
type(numbers)
numpy.ndarray
Do some arithmetic on that array:
numbers + 1
array([1. , 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8, 1.9, 2. ])
Sum up all the numbers:
np.sum(numbers)
np.float64(5.500000000000001)
Some basic graphics with Matplotlib
Matplotlib is the standard package for producing publication-quality graphics, and works hand-in-hand with NumPy arrays.
We usually use the pyplot
submodule for day-to-day plotting commands:
import matplotlib.pyplot as plt
Define some data and make a line plot:
theta = np.linspace(0.0, 360.0)
sintheta = np.sin(np.deg2rad(theta))
plt.plot(theta, sintheta, label='y = sin(x)', color='purple')
plt.grid()
plt.legend()
plt.xlabel('Degrees')
plt.title('Our first Pythonic plot', fontsize=14)
Text(0.5, 1.0, 'Our first Pythonic plot')
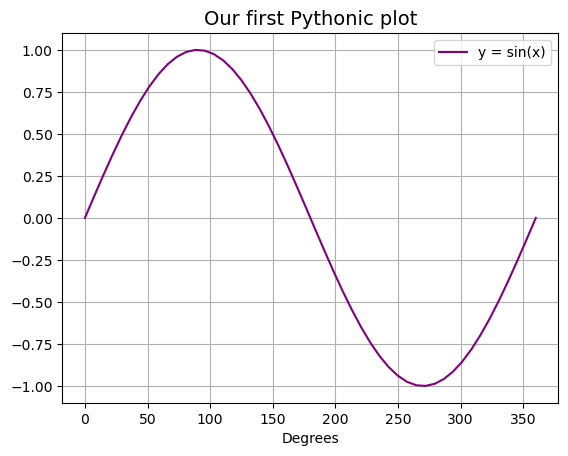
What now?
That was a whirlwind tour of some basic Python usage.
Read on for more details on how to install and run Python and necessary packages on your own laptop.